Eek! Syntax!
Like every full blown language, BASH is syntax sensitive. However, since the syntax is the same as in the normal CLI environment, it isn't that hard to pick up. As a rule of thumb, which isn't an exact science of course, I run commands with the syntax
<do> <switches> <on what>, or for file alterations
<do> <switches> <what> <to where>. This might sound complex at first, but don't fear, once you get your feet wet in CLI and BASH-scripting you'll understand what it means.
However, the following examples will show the syntax of some commands, so you'll probably pick it up quite easily.
What's important for our example is how we run our homemade scripts. There are two options - the first one is to ‘chmod’ the file to be executable and start the script with the
./<script name> syntax. Second, which I usually do, is to execute the script through the standard shell, by issuing
sh <script name>
Basics
The base of every script, much like programming, is variables. Without variables, a script would be a dumb series of commands. Sometimes this is what you desire, but often you require something a bit more intelligent. In spite of the dread that 'programming' and 'variables brings to most non-code-monkeys, using variables is actually quite easy. I’ll use the following script to explain the basics:
#! /bin/bash
echo "What's your name?"
read user_name
echo "Hi ${user_name}!"
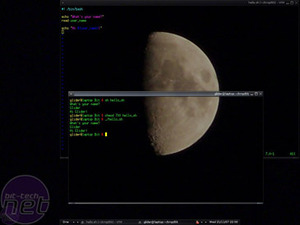
A little less generic than "Hello World!"
Now let’s break it apart line by line:
It all starts with
#! /bin/bash, the line is required to let the interpreter know what to use to run the file. In our case, this is the standard shell, /bin/bash. Next, we ask the user a question, by echoing "What's your name?". We wait for a response and place all the user input in the variable named
user_name with the command
read. Afterwards, we welcome the user by echoing “Hi”, followed by the contents of the variable
user_name, which we call by
${user_name}.
There are, of course, some special variables, some more important than others. The ones that matter to us in this small article are the numbered ones, $1, $2,… These are important because the extra command line options are stored in them. So, when you issue
sh hello.sh Glider, the hello.sh script is executed, and “Glider” is automatically stored in $1.
Another useful thing in lines of source codes are comments. All comments start with “#” and span until the end of the line. One can never comment enough, as it improves the readability to others. Or, if you're anything like Brett, it reminds you what you wrote yourself...
Of course, this is a dumb example, but we have to start somewhere.
Loops
An important thing in computer languages are loops. Loops make the life of a programmer a lot easier - they can potentially save a lot of lines when coding. The two main loops are ‘for’ and ‘while’ loops.
Let’s start with the ‘while’ loop:
#! /bin/bash
n=1
while [ $n -le 10 ]
do
echo $n
((n++))
done
First, we set the variable
n to the value of 1, then we start the loop, which will run while
n is
lower or
equal to 10. And everything between
do and
done will be executed. For this example I’ve taken the value of
n and incremented it by 1, by issuing
((n++)) (those double brackets are important). Lower or equal is one example of a possible
condition - there are
lots more.
Now, on to the ‘for’ loop, which is surprisingly similar to the ‘while’ loop…
#! /bin/bash
for n in `seq 0 10`
do
echo $n
done
There are three differences. First, you don’t need to initialize the variable (
n in this case). Second, you don’t need to increment the variable, because a ‘for’ loop does it for all the values which are given. And third, the line that determines the loop is different. It’s easy though -
for <variable> in <sequence>.
Now, the only weird thing might be that the sequence is generated by
`seq 0 10`, so let's break that part down to the bare essence. ‘Seq’ is a shell command that generates a sequence of numbers.
seq 0 5 creates the sequence 0, 1, 2, 3, 4, 5. But wait - ‘seq’ is a command...how come we can use it in the syntax of the ‘for’ loop? Well, that’s what the ‘`’ (back tick, not single quote) does. Everything placed between back ticks will be run as a command, with the output of the command as the return value. So in essence we typed
for n in 0 1 2 3 4 5 6 7 8 9 10. I don’t know about you, but I prefer the lazy way!
Want to comment? Please log in.